TList's availability is granted by Classes unit recalled with uses command at the top; so we can declare every variable we need:
type pEmployee = ^TEmployee; //Define a poiner of TEmployee TEmployee = Record Id : integer; Name : string; Surname : string; end; type TForm1 = class(TForm) ... private EmployeeList : TList; //list of pointers of employees (list of pEmployee) ... public end;
Look at the comment: list of pointers of employee.
We apparently didn't declare any pointer, and especially any pointer (pEmployee) to TEmployee: in facts we only declared a dynamic array of generic pointers.
Don't be deceived by the name EmployeeList, which is just for coder's comfort; indeed a TList can hosts any pointer, not only the type one we chose: for example we can have a pointer to integer and one to a record in the same List.
That's what generic means! The ability to transform itself into a more specific form.
[Note this concept: we'll recall it inside Object Oriented Programming proposals]
Good, we certainly can start playing with our EmployeeList: but how?
Remember what we wrote before? A TList variable has all functions and procedures needed to manage the dynamic array implicitly declared.
So we just have to access them: but how again?
Well... to be short, this kind of variable is more complex than ones seen until now: for now imagine them like records, with not only other data types but with functions and procedures (called methods) too.
And we know how to get access into a record: so we write EmployeeList followed by a stop and the name of methods.
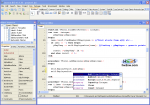
Which are these methods? where can I see them?
Right. You can go here or just wait a second after having put the stop: a little frame will show with all possible voices related to the List. Play with it to see what happens.
[You can find in frame even more items than the more specific ones, and they refer to other construct parents of TList]
Ok... you've seen all methods of TList and you want to add an employee: surely you'll write something like:
EmployeeList.Add(pEmpNew);
After that you keep going on writing code, then compile: by trying to add an employee, the application crashes!